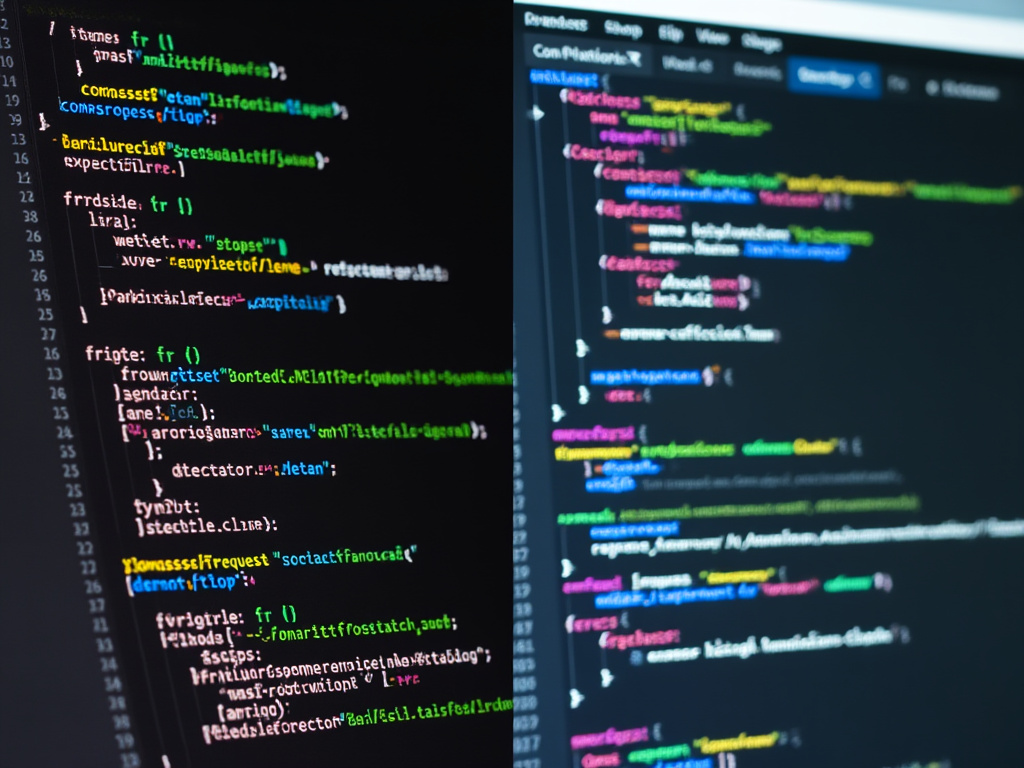
Introduction:
In the evolution of web development, the way we handle asynchronous requests has significantly changed. Two pivotal technologies in this domain are XMLHttpRequest (XHR) and Fetch. While both serve the purpose of making HTTP requests from JavaScript, they offer different approaches, functionalities, and developer experiences. This blog will delve into the differences, advantages, and use cases for each.
1. Background and Evolution:
- XMLHttpRequest:
- Introduced in Internet Explorer 5 in 1999.
- Became the cornerstone for Ajax (Asynchronous JavaScript and XML) applications.
- Initially used for XML but adapted for all types of data.
- Fetch API:
- Part of the modern web standards, introduced by WHATWG.
- Promises-based, making it much simpler to deal with asynchronous operations.
2. Syntax and Usage:
- XMLHttpRequest:
var xhr = new XMLHttpRequest(); xhr.open('GET', 'url', true); xhr.onload = function () { if (xhr.status == 200) { console.log(xhr.responseText); } }; xhr.send();
- Fetch API:
fetch('url') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
3. Key Differences:
- Promises vs Callback: Fetch uses Promises, providing a cleaner, more manageable way to handle asynchronous operations compared to the callback-driven XHR.
- Default Headers: Fetch doesn’t send cookies unless credentials: ‘include’ is set, whereas XHR does. This can be a security and performance feature.
- Streaming: Fetch supports streaming responses, allowing for processing data as it arrives, which can be beneficial for large downloads or real-time data.
- Error Handling: Fetch will only reject on network errors, not HTTP errors like 404 or 500, whereas XHR considers these as successful if the server responds.
- Request Configuration: Fetch offers a more straightforward way to set headers, methods, and body through an options object.
4. Advantages of Fetch:
- Simpler Syntax: Easier to read and understand due to its promise-based nature.
- Modern Web Standards: Aligns better with current JavaScript practices like async/await.
- Built-in Response Handling: More intuitive handling of different response types (JSON, Blob, ArrayBuffer).
5. When to Use Each:
- XMLHttpRequest:
- Legacy systems or libraries that might not support Fetch.
- When you need fine-grained control over the request that isn’t covered by Fetch’s simpler API.
- Fetch:
- Modern web applications where you want to leverage promises or async/await.
- Any scenario where simplicity, readability, and modern JavaScript practices are prioritized.
6. Browser Support:
- Fetch has excellent modern browser support but might require a polyfill for older browsers.
- XHR is universally supported but might lack some newer features found in Fetch.
Conclusion:
Choosing between Fetch and XMLHttpRequest largely depends on your project’s needs, the complexity of your HTTP interactions, and the coding environment you’re working in. Fetch provides a modern, promise-based approach that aligns well with contemporary JavaScript practices, making it preferable for new projects. However, XHR’s broad support and traditional usage still make it relevant, especially in maintaining or updating legacy systems.