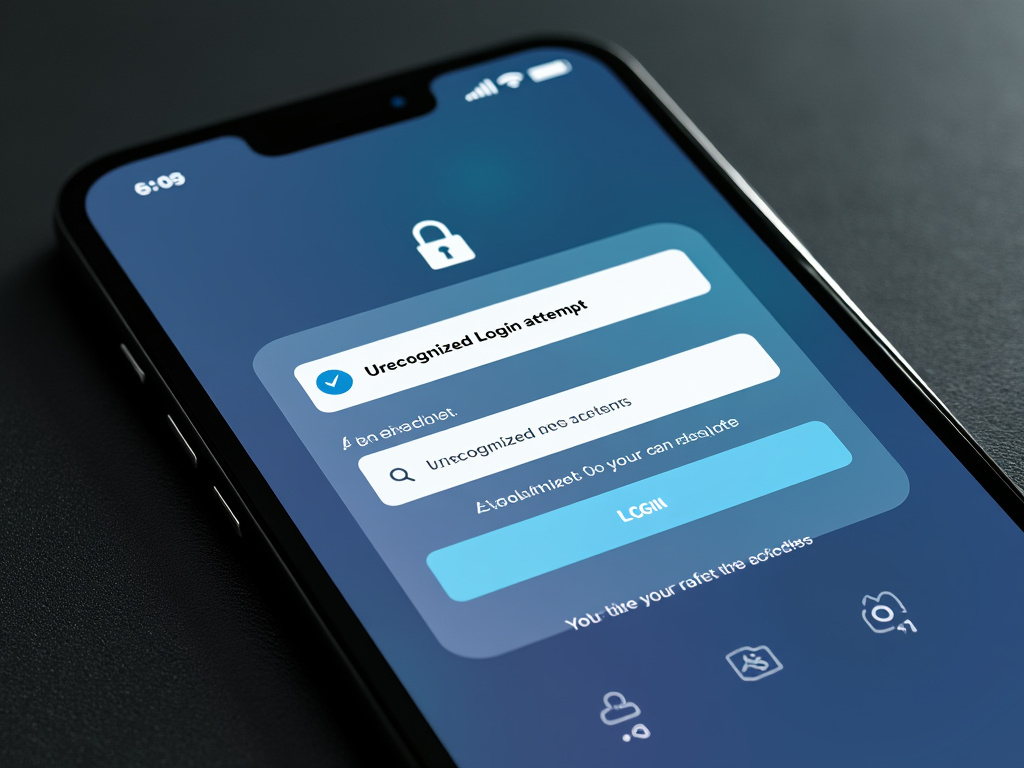
Introduction:
In today’s digital landscape, security is paramount. As a developer, one way you can bolster user security is by implementing unrecognized device login alerts. This feature notifies users when their account is accessed from a new or unrecognized device, offering an additional layer of protection against unauthorized access. Here’s how you can integrate this into a React application.
Why Implement Unrecognized Device Alerts?
- Security: Alerts can prompt users to verify new login attempts, potentially stopping attackers in their tracks.
- User Trust: It reassures users that their account’s integrity is being monitored.
- Transparency: Users are kept in the loop about their account activity, especially relevant in shared or public device usage scenarios.
Step-by-Step Implementation:
1. Setting Up the Project:
- Ensure you have a React project. If not, initialize one with npx create-react-app my-app.
- Install necessary dependencies like axios for HTTP requests:
bashnpm install axios
2. Creating User Device Recognition:
- Frontend Logic:
- Use react-device-detect to gather device information like browser type or device type.
javascriptimport { isMobile, browserName } from 'react-device-detect'; const deviceInfo = { isMobile: isMobile, browser: browserName, // Add more device info as needed }; // Send this info to backend on login
- Backend Integration:
- Your server should store this device info against user profiles. When a login occurs, compare new device info with stored data.
3. Implementing Alerts:
- Backend Alert Logic:
- On login, if the device isn’t recognized:
- Trigger an alert via email or push notification.
- On login, if the device isn’t recognized:
4. React Component for Login:
jsx
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const Login = () => {
const[error, setError] = useState('');
const[email, setEmail] = useState('');
const[password, setPassword] = useState('');
useEffect(() => {
// Check for security alerts on component mount
const checkForAlerts = async () => {
try {
const alerts = await axios.get('/api/check-login-alerts');
if (alerts.data.unrecognized) {
alert("Your account was accessed from an unrecognized device recently. Please verify it's you.");
}
} catch (error) {
console.error("Error checking for alerts:", error);
}
};
checkForAlerts();
}, []);
const handleSubmit = async (event) => {
event.preventDefault();
try {
const response = await axios.post('/api/login', {
email,
password,
deviceInfo: {
isMobile: isMobile,
browser: browserName,
}
});
if (response.data.success) {
// Successful login, redirect or update state
} else {
setError(response.data.message);
}
} catch (err) {
setError('Login failed. Please try again.');
}
};
return (
<form onSubmit={handleSubmit}>
<input type="email" value={email} onChange={e => setEmail(e.target.value)} placeholder="Email" required />
<input type="password" value={password} onChange={e => setPassword(e.target.value)} placeholder="Password" required />
<button type="submit">Login</button>
{error && <p>{error}</p>}
</form>
);
};
export default Login;
5. Backend (Example with Node.js & Express):
javascript
app.post('/api/login', (req, res) => {
const { email, password, deviceInfo } = req.body;
// Validate user & password here, then:
User.findOne({ email }, (err, user) => {
if (err || !user) return res.json({ success: false, message: 'User not found' });
if (user.knownDevices.findIndex(d => d.browser === deviceInfo.browser && d.isMobile === deviceInfo.isMobile) === -1) {
// Device not recognized, send alert
sendAlert(email, 'Login from an unrecognized device detected!');
user.knownDevices.push(deviceInfo);
user.save();
}
// If login successful
res.json({ success: true, message: 'Logged in successfully' });
});
});
function sendAlert(email, message) {
// Implement sending email or push notification
}
Challenges and Considerations:
- Privacy: Ensure you’re not collecting too much personal information.
- False Positives: Users might often change devices; consider user feedback mechanisms to manage false alarms.
- Security: Use HTTPS for all communications involving sensitive data.
Conclusion:
Implementing unrecognized device login alerts in React not only enhances security but also builds trust with your users. This guide provides a basic framework, but remember, security is an ongoing process. Regularly update your methods and stay informed about new vulnerabilities and solutions.