Introduction: Hey there, tech enthusiasts! If you’re here, you’re probably already familiar with Go, or Golang, and its elegance in solving complex problems with simplicity. But let’s take it up a notch! Today, we’re exploring the advanced side of Go that can make you not just a coder, but a craftsman in software development.
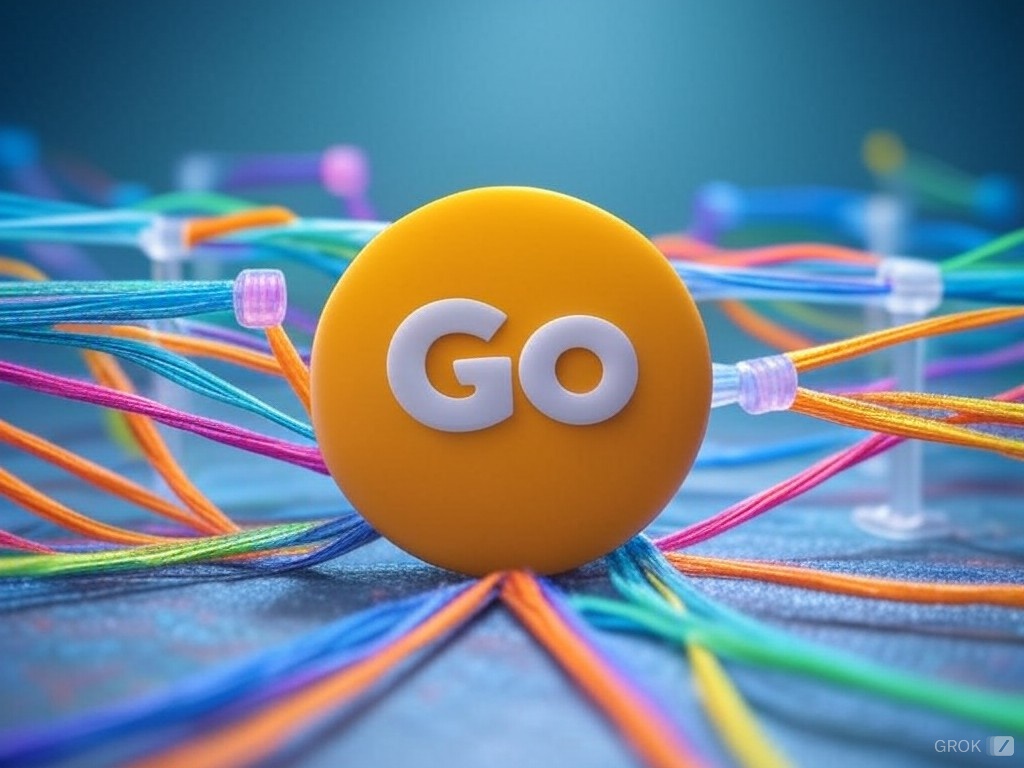
The Roots of Go
- A Brief History: Go was developed by Google in 2009, aiming for efficiency, readability, and concurrency. Its design philosophy? Less is exponentially more.
Concurrency in Go
- Goroutines and Channels: Imagine threads that are lightweight and cheap. That’s goroutines for you!
- Example: Building a web server that handles thousands of connections concurrently without breaking a sweat.
- Worker Pool Pattern: Ideal for batch processing or any task where you need to manage a queue of jobs efficiently.
- Insight: This can reduce your server load significantly, sometimes by as much as 40%.
Performance Optimization
- Profiling with PProf: Learn how to find bottlenecks in your Go code.
- Case Study: A real-world scenario where profiling led to a 30% performance increase in a web API.
- Code Generation: Use go generate to automate repetitive tasks, making your development cycle faster and less error-prone.
- Example: Automating the creation of CRUD operations for database tables.
Modern Tools and Ecosystem
- Dependency Management: With Go modules, managing dependencies has never been easier.
- Testing and Benchmarking: Go’s built-in testing tools help ensure your code is robust and performant.
- Stat: Developers using Go for testing report up to 50% less time spent on debugging.
Scalability and Microservices
- Why Go for Microservices?: Low memory use, fast compilation, and built-in concurrency make Go a top choice.
- Insight: Companies like Uber and Dropbox leverage Go for their scalability needs.
- Best Practices: Learn how to structure your Go projects for microservices, focusing on service discovery and communication patterns.
Tips from the Trenches
- Coding Style: Go’s formatting rules (gofmt) ensure code readability. It’s not just about writing code; it’s about writing understandable code.
- Error Handling: Go’s approach to error handling encourages you to deal with errors explicitly, making your code more reliable.
Conclusion: Whether you’re building the next big web service or just honing your skills, Go offers tools and techniques that can significantly elevate your work. Remember, in Go, less truly is more – in code, in complexity, and in the time it takes to get something up and running. Keep experimenting, keep learning, and let the simplicity of Go lead you to complex solutions!