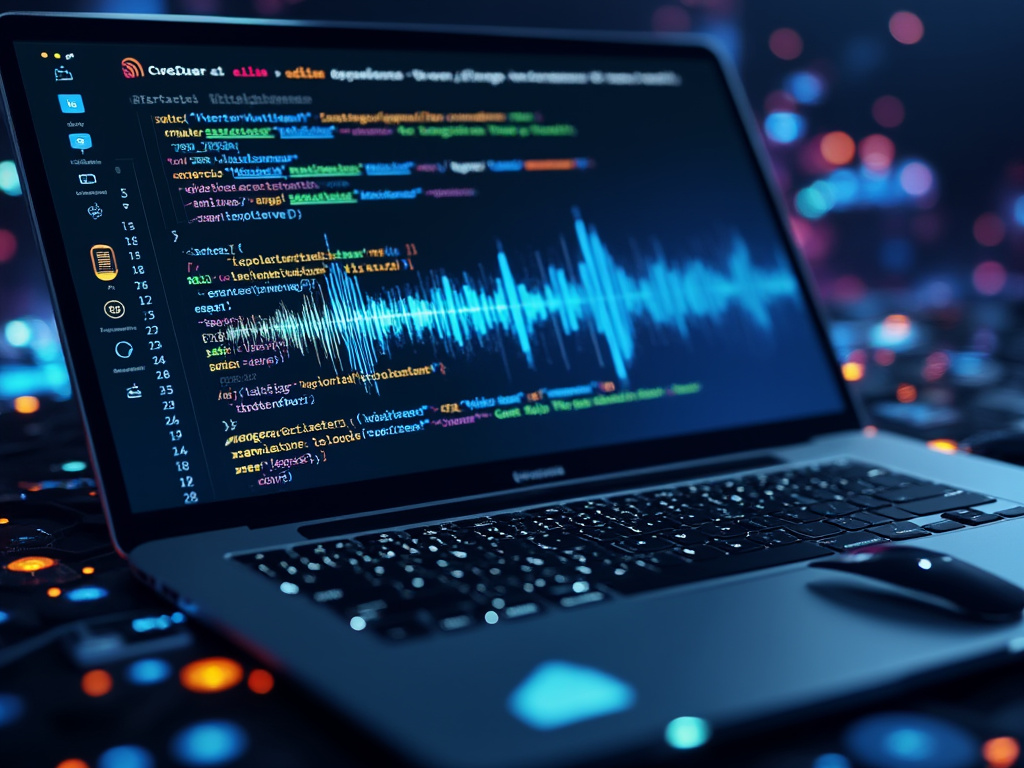
Are you ready to build your own audio transcription app? In just a few steps, you can put together a powerful tool using VZ’s slick UI components, integrate Gro’s transcription engine with Whisper, and streamline your workflow with the help of Cursor.
In this post, we’re going to walk through the entire process. From setting up the environment to testing it with a real audio file, you’ll learn how to create an app that accepts an audio input and returns a fully transcribed text. Let’s not waste a second. Here’s how you can do it with VZ, Gro, and Cursor.
Overview of the Process
In this guide, we’ll build an audio transcription app using:
- VZ: A solution for creating UI components fast.
- Cursor: A tool that speeds up your coding process with features like auto-completion and documentation integration.
- Gro: A robust audio transcription service using OpenAI’s Whisper models.
- Next.js: A popular React framework you’ll use to structure your code.
We’ll walk through initializing the project, bringing in UI components, configuring the backend to work with AI transcription, and making everything look seamless. Let’s dive in.
Setting Up the Environment
Getting to Know The Tools
Before we get started, let’s get acquainted with the tools we’ll be using:
- VZ: Known for its ability to create beautiful React-based UI components quickly, saving both time and headaches.
- Gro: Provides state-of-the-art transcription capabilities through Whisper by OpenAI. If you need to convert audio into text accurately, Gro is your go-to tool.
- Next.js: This framework is the backbone of our application. It allows for server-side rendering and delivers excellent performance out-of-the-box.
- Cursor: It’s like your smart coding companion. From auto-suggestions to adding external documentation, Cursor makes everything smoother as you build.
Installing the Necessary Software
Let’s get the basic setup out of the way before the fun starts.
- Ensure you have bun.js installed by running:
curl -fsSL https://bun.sh/install | bash
- Create a new Next.js project using Bun’s shortcut command
bun x
:bun x create next-app transcription-app
- Once created, jump into your project folder:
cd transcription-app
- Now, install VZ. This is where the magic begins. VZ will handle the UI for receiving audio files and displaying transcriptions later on. Grab the command for VZ’s audio transcription component:
bun add @vz/transcription
That’s the dry setup stuff out of the way. Now let’s get to building.
Creating the Initial UI with VZ
Designing an Audio Transcription Interface
The interface, at least the user-facing part, should be simple. All you need is an area for users to upload an audio file and a space on the right to display the transcription after processing. It sounds basic, and with VZ, it can be set up in minutes.
The key component we’ll use here is VZ’s Audio Transcription Component. It includes:
- File Input: Where users will upload their audio files.
- Transcription Output: A space to display the text transcriptions once processing completes. The cool part? You can download the result with a click.
Implementing the UI Component
- First, import the UI component into your project. Here’s the command:
bun add @vz/audio-transcription
- Now include it in your Next.js project by modifying the homepage file (
pages/index.js
). Add the transcription component where you’d like it to appear on the page.
Thanks to VZ, the UI gets built in a flash! All that’s left is to wire it to handle the actual transcription.
Setting Up a New Next.js Project
You’re already partly there since we’ve got the skeleton of the Next.js app. Here’s a little more detail on how to get everything working perfectly.
Initiating the Project
You used bun x create-next-app
earlier to create the folder structure for your app. Now, we’ll tackle the directory structure and put everything in order so that Next.js is aware of our component.
Take a look inside your pages
folder. That’s where you’ll place your main logic. You can structure it for clean separation between your frontend (the VZ UI) and backend (our transcription engine using Gro.)
Integrating VZ Components
There’s a slight learning curve when working across two frameworks: VZ’s UI and Next.js. One issue that may pop up is class interference from Next’s templates. If that happens:
- Open your style sheet (or create one), and tweak the styling to ensure there aren’t any conflicts.
Once those adjustments are in place, you’ll be staring at a clean, modern UI, ready to accept audio files.
Enhancing the Application with Cursor
Time to bring Cursor into the fold. Cursor does two things well: code completion and keeping your project organized with documentation.
Leveraging Auto-Completion
As you’re developing, Cursor will kick in and help you autocomplete repetitive code patterns, especially when setting up things like routes, initializing variables, or bootstrapping components.
In this case, you’ll notice it comes particularly handy when connecting to the Gro API.
Integrating Documentation
Cursor allows you to add external documentation directly to your project. For instance, we’re going to use Gro’s Whisper API for transcription. Add its documentation to Cursor by including the link:
- Open up Cursor, go to the docs section, and simply paste the link to Whisper (Gro) documentation.
Cursor will index and make that part of your coding environment—so you can look it up whenever you’ll need to reference an API call.
Connecting to Gro for Transcription
It’s time to wire this baby up for transcription.
Sending Audio Files to Gro
To process audio, we’ll send the uploaded audio files to Gro. Written in OpenAI’s Whisper, Gro provides models that can transcribe various languages and accents with minimal error.
Here’s the high-level process:
- An audio file is uploaded via the UI.
- The request is passed to Gro’s API endpoint.
- Gro processes the audio and returns the transcribed text to be displayed in the UI.
Configuring Environment Variables
Gro requires a few environment configurations—you’ll need your API key and Gro’s base URL. The best practice in Next.js is to save these keys in a .env.local
file.
Create .env.local
, and add these variables:
GROQ_BASE_URL=https://groq.io/api/whisper
GROQ_API_KEY=[your-api-key-here]
Some additional setup:
- Install the OpenAI SDK to handle the connection:
bun add openai
- Now modify
pages/api/transcribe.js
to make the API call. Use the Whisper Large model provided by Gro for transcription accuracy. - Have your app send the uploaded audio file to Gro’s API, get the response, and output the transcription into your UI.
Building and Testing the Application
With things almost set up, it’s time to test the app to make sure everything works together.
Creating an Audio File with 11 Labs
Before we test, we need audio. 11 Labs makes it easy to generate sample audio for testing purposes:
- Head to 11 Labs and create a simple file. For example, generate a speech saying: “Hello world, this transcription test is successful!”
- Download the file—you’ll use this during testing.
Testing the Transcription Process
- Open your running Next.js app.
- Use the file selector on the UI (created by VZ) to upload the audio file you just downloaded.
- Click transcribe and wait.
You should see the transcribed text on the right side of the screen.
If everything works, you have your own transcription app! Notice how quickly it transcribes and how accurate the Whisper model is.
Exploring Additional Features and Enhancements
Composer’s Capabilities
One underappreciated tool we used is Composer. Here’s the deal:
Rather than creating files manually, Composer handles that for you. When the time came to create the .env
file, Composer auto-generated the file structure and routes for us.
If you’re building out complex apps, this could save hours by keeping your project organized at all times.
Logging Application Performance
Don’t forget to do ample logging during development. You can use console.log
on the backend to track what your app is doing. Check for errors, make sure your API calls to Gro are working as expected, and adjust as needed.
Conclusion
We’ve just walked through the entire process of building a transcription app with VZ, Gro, and Cursor. From setting up the UI to handling audio transcription and configuring your environment for success, everything has been covered.
With a bit more time, you can add more features: maybe support for multiple languages, real-time transcription, or even voice commands. But for now, you’ve got the framework for a powerful, working transcription tool.
Don’t stop here—take this project further. The potential for enhancements is limitless.