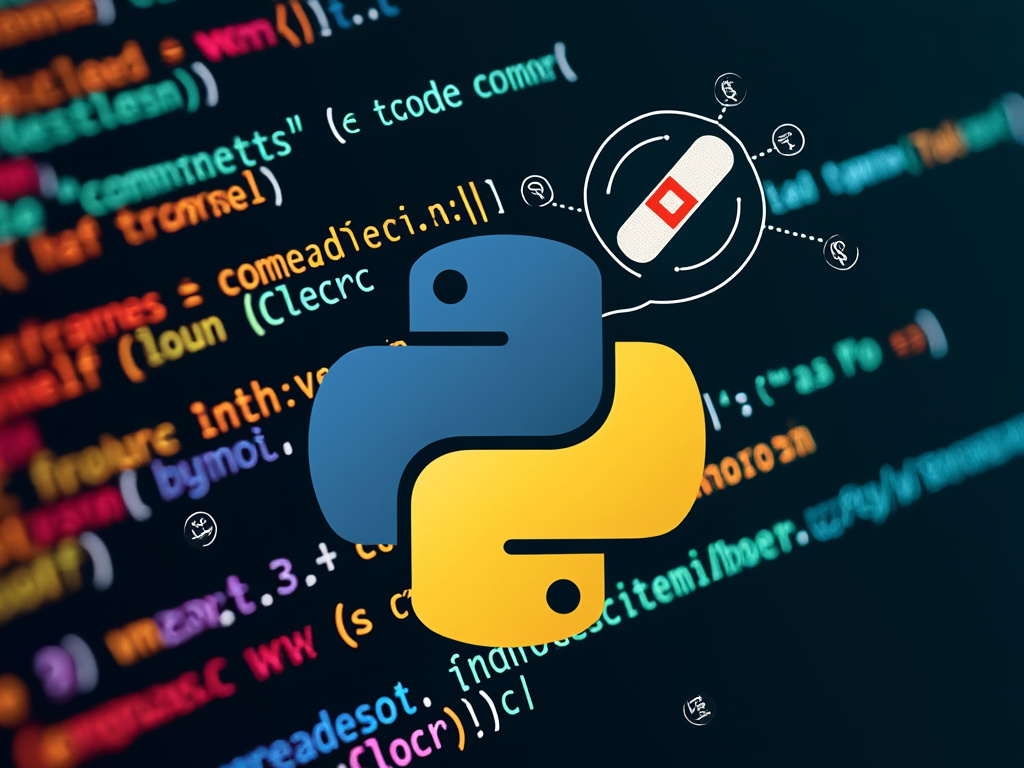
Introduction:
In the world of software development, handling production exceptions can often feel like fighting a hydra — cut one off, and two more appear. The OpenExcept project introduces “SelfHeal,” an innovative approach where code attempts to fix its own exceptions. This blog dives into the concept, its implementation, and the implications for developers and operations personnel.
Understanding SelfHeal:
SelfHeal is not about preventing exceptions but about autonomously resolving them once they occur. Here’s how it works:
- Exception Detection: When an exception occurs, SelfHeal analyzes it in real-time.
- Pattern Recognition: It uses historical data to recognize patterns or common issues that lead to exceptions.
- Automated Correction: Based on pre-defined logic or machine learning models, SelfHeal attempts to correct the issue, like modifying configurations or data.
Why Can’t Code Fix Exceptions Itself?
- Complexity: Many exceptions arise from complex interactions, data anomalies, or external system failures which aren’t always straightforward to fix programmatically.
- Context: Without understanding the broader context or business logic, automatic fixes might lead to unforeseen consequences.
- Security: Automatic code or configuration changes can introduce vulnerabilities if not carefully managed.
- Ethics and Accountability: There’s a responsibility aspect where human intervention is needed for significant system changes.
Target Audience:
- DevOps, Production Engineers: They benefit from reduced downtime and fewer manual interventions.
- Software Engineers: Especially those in roles dealing with frequent escalations, SelfHeal promises to lessen the burden of exception handling, allowing focus on development rather than maintenance.
How SelfHeal Works – A Technical Overview:
- Installation: Integrated into your Python application, SelfHeal hooks into your error handling mechanisms.
- Exception Logging: Every exception triggers a log event, which SelfHeal uses for analysis.
- Healing Logic: python code !
from selfheal import SelfHeal self_healer = SelfHeal(service_name='YourService') @self_healer.register_healer def handle_division_by_zero(exc, *args, **kwargs): # Example healing logic if isinstance(exc, ZeroDivisionError): return 0, True # Returns a value to replace the result and True to indicate healing was attempted return None, False # No healing, exception continues to propagate # Usage in your code try: result = 10 / 0 except Exception as e: healed_value, healed = self_healer.heal(e) if healed: print(f"Healed value: {healed_value}") else: print(f"Exception not healed: {e}")
- Healing Logic: python code !
Current Limitations:
- Language Support: Currently only Python is supported, with plans to expand to other languages.
- PR Automation: It doesn’t yet open Pull Requests automatically for code fixes, which would be a valuable addition for version control systems.
Live Demos and GitHub Integration:
- GitHub Page: Visit OpenExcept/SelfHeal for live demos, GIFs showing the healing process, and to see the project in action.
- Community Contributions: The open-source nature allows developers to contribute, report issues, or suggest improvements.
Future Prospects:
- Expanded Language Support: Plans to include support for languages like Java, JavaScript, and C#.
- Advanced AI: Incorporating more sophisticated AI to predict and prevent exceptions before they occur.
- Integration with DevOps Tools: Enhancing its capabilities to work seamlessly with CI/CD pipelines, monitoring tools, and ticketing systems.
Conclusion:
While SelfHeal isn’t a silver bullet for all production issues, its approach to autonomous exception handling is a step forward in making systems more resilient and self-sufficient. It represents a paradigm where software can not only detect its own faults but also attempt to rectify them, potentially revolutionizing how we manage software reliability.